There are many ways to get array of values from array of objects in Javascript. You can use the map()
method and create a function to get array of values. Let’s learn about it with the explanation and examples below.
Get array of values from array of objects in Javascript
Method 1: Use the map() method
We can get array of values from array of objects by using the map()
method in Javascript.
Syntax:
array.map(function(currentValue, index, arr), thisValue)
Parameters
- function(): Run through all the elements of the array
- currentValue: The value of the current element.
- index: Index of the element.
- arr: Array of the element.
- thisValue: Value passed to the function, normally default value
undefined
.
Return Value: A new array.
Example 1 :
// Creating a function that calculates the square of a number function pow(number) { return Math.pow(number, 2); } const array = [2, 4, 6, 8]; // You can calculate the square of all the elements in this array by using the map() method and calling the pow() function const powArr = array.map(pow); console.log("List of numbers after squared"); // Expected output of new array is [4, 16, 36, 64] console.log(powArr);
Output
List of numbers after squared
[4, 16, 36, 64]
Example 2:
const us_president = [ {first_name : "Donal", last_name: "Trump"}, {first_name : "Barack", last_name: "Obama"}, {first_name : "Joe", last_name: "Bidden"}, {first_name : "Abraham", last_name: "Lincoln"} ]; // Creating a function to get full name of us_president by using join() method function getFullName(name) { return [name.first_name, name.last_name].join(" "); } const full_name = us_president.map(getFullName); console.log("List of presidents of the United States of America") console.log(full_name);
Output
List of presidents of the United States of America
["Donal Trump","Barack Obama","Joe Bidden","Abraham Lincoln"]
Example 3
const presidents = [ { name: "Obama", age: 61}, { name: "Trump", age: 73}, { name: "Biden", age: 80} ]; // We can get the name of presidents by using syntax of map() method let getName = presidents.map(({ name }) => name); // Print out the name of presidents console.log("List name of Presidents is"); console.log(getName);
Output
List name of Presidents is
["Obama","Trump","Biden"]
Method 2: Create a function to get array of values
Example
const presidents = [ { name: "Obama", age: 61}, { name: "Trump", age: 73}, { name: "Biden", age: 80} ]; // Creating a function to get name of presidents function getName(array, input) { var listName = []; // Loop over all element of 'presidents' , if value is name, we put it to new array 'listName' for (var i = 0; i < array.length; ++i) { listName.push(array[i][input]); } // The return value is a list return listName; } var result = getName(presidents, "name"); console.log("List name of Presidents is") console.log(result)
Output
List name of Presidents is
["Obama","Trump","Biden"]
Summary
Our tutorial has guided you through a way to get array of values from array of objects in Javascript by using the map()
method and creating a function to get array of values. We always hope this tutorial is helpful to you. If you have any questions, please let us know by leaving your comment in this tutorial. Thank you.
Maybe you are interested:
- Get the min/max elements of an array in javascript
- Get an Object’s Keys as Array in JavaScript
- Get Difference between two Arrays of Objects in JavaScript
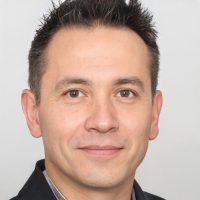
My name is Fred Hall. My hobby is studying programming languages, which I would like to share with you. Please do not hesitate to contact me if you are having problems learning the computer languages Java, JavaScript, C, C#, Perl, or Python. I will respond to all of your inquiries.
Name of the university: HUSC
Major: IT
Programming Languages: Java, JavaScript, C , C#, Perl, Python